Definition: The Fibonacci sequence is defined by the equation, where \(F(0) = 0 \), \(F(1) = 1 \) and \(F(n) = F(n-1) + F(n-2) \text{for } n \geq 2 \). This gives us the sequence 0,1,1,2,3,5,8,13 … called the Fibonacci Sequence. This post is about how fast we can find the nth number in the […]
Fast nth Fibonacci number algorithm
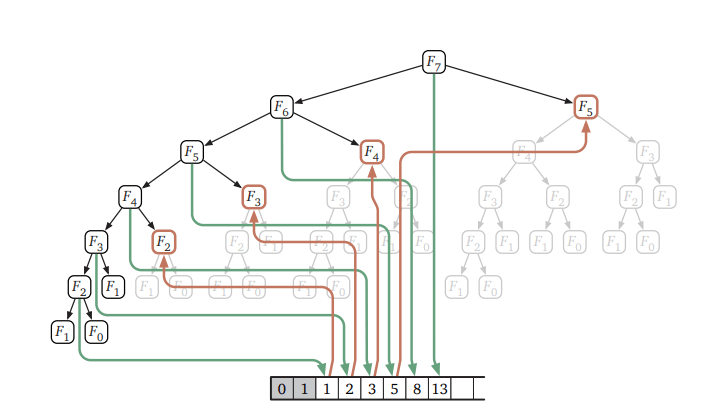