Install supervisor
# update apt
sudo apt-get update -y
# install supervisor
sudo apt-get install supervisor -y
# check if supervisor is running fine
sudo systemctl status supervisor
Enable Supervisor Web Interface
# edit supervisor configuration file
sudo nano /etc/supervisor/supervisord.conf
Add the below line in the end of supervisor.conf file
[inet_http_server]
port=*:9001
username=admin
password=admin
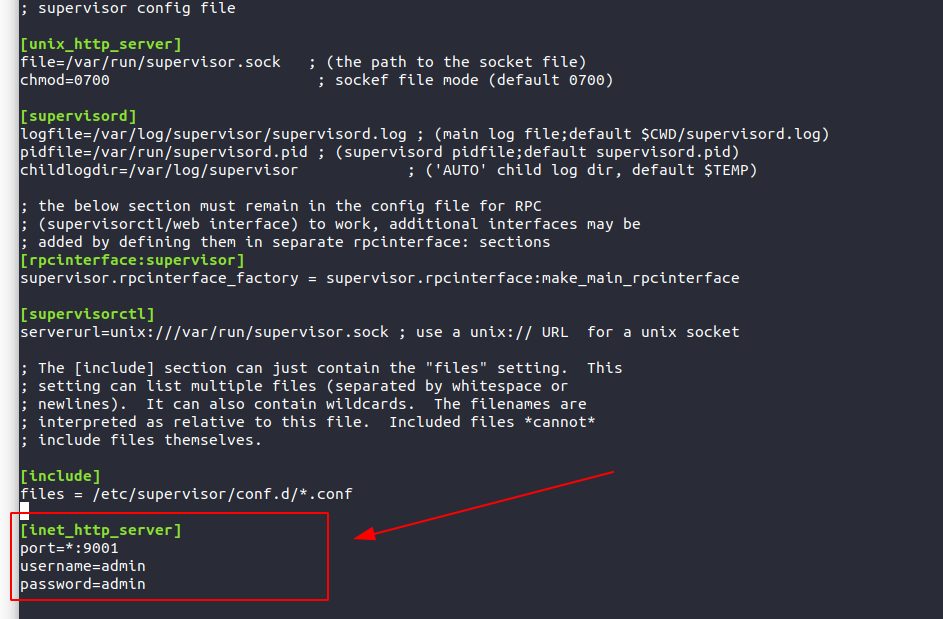
# restart supervisor to see your conf changes
sudo systemctl restart supervisor
Install Jupyter lab
Check the official website for latest installation instructions. Below line is what I used.
# run one of the below installation PIP
pip install jupyterlab
# or
pip3 install jupyterlab
Run jupyter lab
jupyter lab
If you get an error that this command is not found, then you probably need to add this command to your system PATH
Edit the bashrc file
nano ~/.bashrc
Add the below line to the end of this file
export PATH="$HOME/.local/bin:$PATH"
source ~/.bashrc
Now try running jupyter lab command again, it should work fine
Installing Jupyter as a service
Create a conf file inside supervisor conf directoruy
sudo nano /etc/supervisor/conf.d/jupyter.conf
Paste the below code in the jupyter.conf file
[program:jupyter]
command=/home/panther/.local/bin/jupyter lab --port 5000 --no-browser --ip="0.0.0.0" --NotebookApp.token='' --NotebookApp.password=''
user=panther
autostart=true
autorestart=true
startretries=5
numprocs=1
startsecs=0
process_name=%(program_name)s_%(process_num)02d
stderr_logfile=/var/log/supervisor/%(program_name)s_stderr.log
stderr_logfile_maxbytes=10MB
stdout_logfile=/var/log/supervisor/%(program_name)s_stdout.log
stdout_logfile_maxbytes=10MB
NOTE:
- change the
user
value - add a password to
--NotebookApp.password
- change the home directory in command, mine is panther so it is /home/panther. Yours is most likely
ubuntu
Access the app from browser
http://<IP>:5000/lab
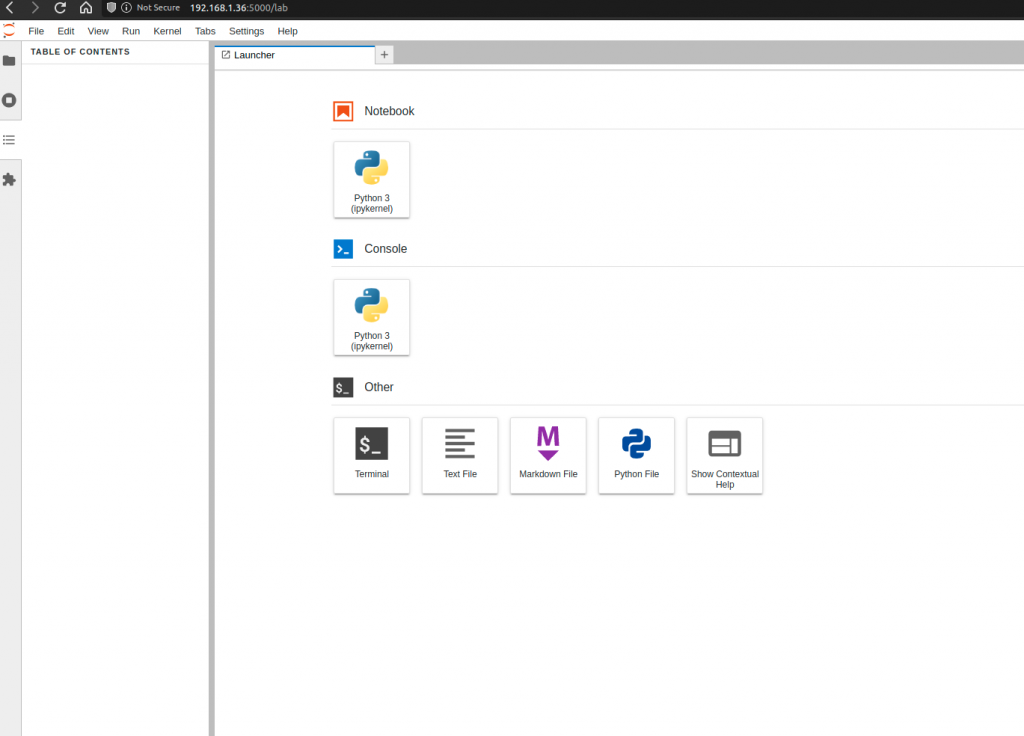