Inheritance is a concept which says you can create a class from another class if you would like to use its properties.
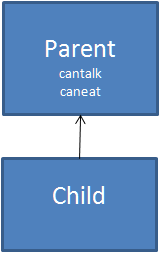
A parent cantalk and caneat, so can the child because child “is a” subclass of Parent.
While most programming languages have the concept of classes, in javascript its all about objects inheriting from other objects. With ECMAScript 6 you can also create classes using the class keyword. But in this post we will only talk about prototypical inheritance.
Creating Inheritance using ___proto___
__proto__ is a special property in an object where when a property is accessed and the interpreter can’t find it in the object, it follows the __proto__ link and searches it there.
var parent = { caneat : true, cantalk : true } var child = { } child.__proto__ = parent; //inheriting from parent console.log(child.caneat);//prints true child.hasOwnProperty(caneat); //this throws error because caneat is a property of the parent
The code above shows basic inheritance where all the properties available in parent is also a part of child. What we did was set the __proto__ variable of object child to parent to let the interpreter look into the parent if the looked up keys are not found in child. But if I change the value of caneat in the parent will the child be effected?
parent.caneat = false; console.log(child.caneat); //yes this returns false.
What if I add another property to our parent?
parent.canwalk = true; console.log(child.canwalk); //yes this returns true.
__proto__ is not the most preferred way of achieving inheritance because its not cross browser friendly and will work only in Chrome and Firefox. Another way of creating subclass is by using Object.create which does the exact same thing that __proto__ does but without the use of __proto__ keyword.
Creating Inheritance using Object.create
var parent = { caneat : true, cantalk : true } var child = { } child = Object.create(parent); //inheriting from parent console.log(child.caneat);
child = Object.create(parent);
is exactly the same as child.__proto__ = parent;
There is a better way of creating subclasses which work across browsers. This method uses .prototype.
Creating Inheritance using prototype
When using .prototype, the dynamics of achieving inheritance changes a bit because you need to create a class for it.
var parent = { caneat : true, cantalk : true } var Child = function(){ //creating a class named Child } Child.prototype = parent; //inheriting from parent var child = new Child(); console.log(child.caneat);//returns true
The above example is the simplest form of prototype inheritance as the parent is an object and the child is a function with no parameters but this is not the ideal scenario because we would be creating Objects of Functions and inheriting them most of the time.
inheritance in non parameterized contructors
// Define the Parent constructor var Parent = function(){ this.caneat = true; this.cantalk = true; } // Define the Child constructor var Child = function(){ Parent.call(this);//the most important part of code } Child.prototype = Object.create(Parent); //inheriting from parent var child = new Child(); console.log("Can child also eat? " + child.caneat);//this returns true
Did you see the use of call method in our Child class. This is similar to using the super keyword in Java without which you cannot inherit the Parent. call method ensures that the Parent object instance is created for the child to inherit otherwise there is nothing given to the child.
inheritance in parameterized contructors
var Parent = function(caneat,cantalk){ this.caneat = true; this.cantalk = cantalk } // Defining a class constructor with NO parameters var Child = function(){ Parent.call(this);//I am not setting the parent variables } Child.prototype = Object.create(Parent); //inheriting from parent var child = new Child(); console.log("Can child also eat? " + child.caneat);//this is undefined
The above code returns the value of caneat to be undefined because the value has not been passed to the Parent from its constructor call. See another version of the same code below.
var Parent = function(caneat,cantalk){ this.caneat = caneat; this.cantalk = cantalk } var Child = function(caneat,cantalk){ Parent.call(this,caneat,cantalk);//passing all variables } Child.prototype = Object.create(Parent); //inheriting from parent var child = new Child(true,true); console.log("Can child also eat? " + child.caneat);//this is true
Now we are setting the values of our parent from our child from its constructor and thus we are able to read the values of parent properties from the child object.
Private and Public variables
Any time you talk about inheritance in any programming language, there are these two access specifiers which never go ignored. Some properties or methods must remain private to the parents and should not be allowed to be inherited by the child. You can achieve it by using the var keyword to initialize your variable instead of this. See the code below.
var Parent = function(caneat,cantalk){ var caneat = caneat; //this is a private variable this.cantalk = cantalk; //this is a public variable } var Child = function(caneat,cantalk){ Parent.call(this,caneat,cantalk); //calling the parent constructor } Child.prototype = Object.create(Parent); //inheriting from parent var child = new Child(true,true); console.log(child.caneat);//this is undefined
The caneat variable in this case remains undefined because the parent has not exposed that property for inheritance.
Thats all about javascript inheritance!