Different ways of setting a value to a Javascript object
//create an object var myVar = {}; //1. set value using dot myVar.key = "myvalue"; //2. set value using square brackets myVar["key2"] = "myValue2" //3. set value using defineProperty Object.defineProperty(myVar,"key3",{ value : "myValue3" });
Setting values using the dot syntax or square brackets is pretty easy to understand but using defineProperty seems unusual. As you can see in the code, the defineProperty function of Object takes three parameters.
Object.defineProperty(obj, prop, descriptor)
obj - The object variable prop - the name of the property that needs to be updated in our variable descriptor - this parameter helps us in making our object immutable or mutable.
The descriptor is an object that can take four parameters
Object.defineProperty(obj, 'key', { enumerable: false, //default is false configurable: false, //default is false writable: false, //default is false value: 'static' });
About writable
When you set a value to a property using defineProperty, you are making it an immutable property, i.e you cannot change its value. Take a look at the snap below.
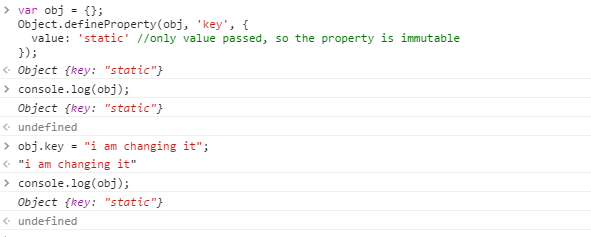
As you can see, the value of key never changes even when I try to set it explicitly.
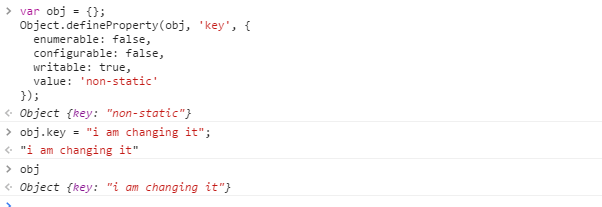
When i make writable to true, the object key behaves like a normal key which can be changed.
About enumerable
Enumerable simply means “can be looped”. This property is used to restrict the set property to show up when using the for…in loop.
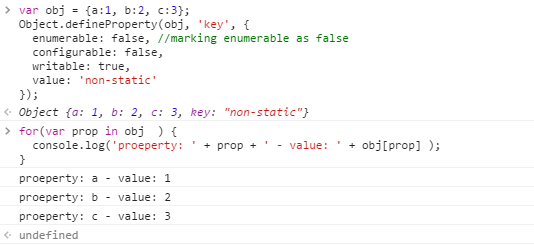
As you can see in the code above, our property “key” is enumerable.
About Configurable
Configurable restricts any change to the property, like deleting or changing its behaviour except writable.
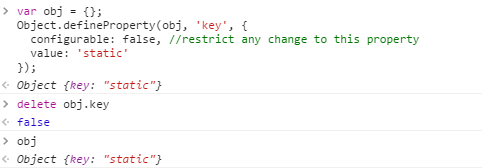
When configurable is false, I cannot delete the property from my object.
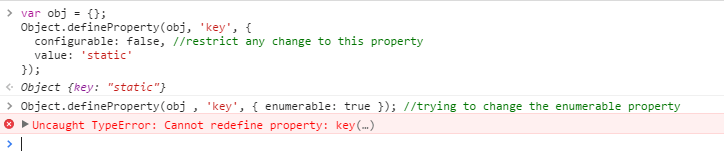
The code throws exception if I try to change the property’s enumerable behavior.